37 Common JavaScript Utility Functions for String, Object, Array & Date
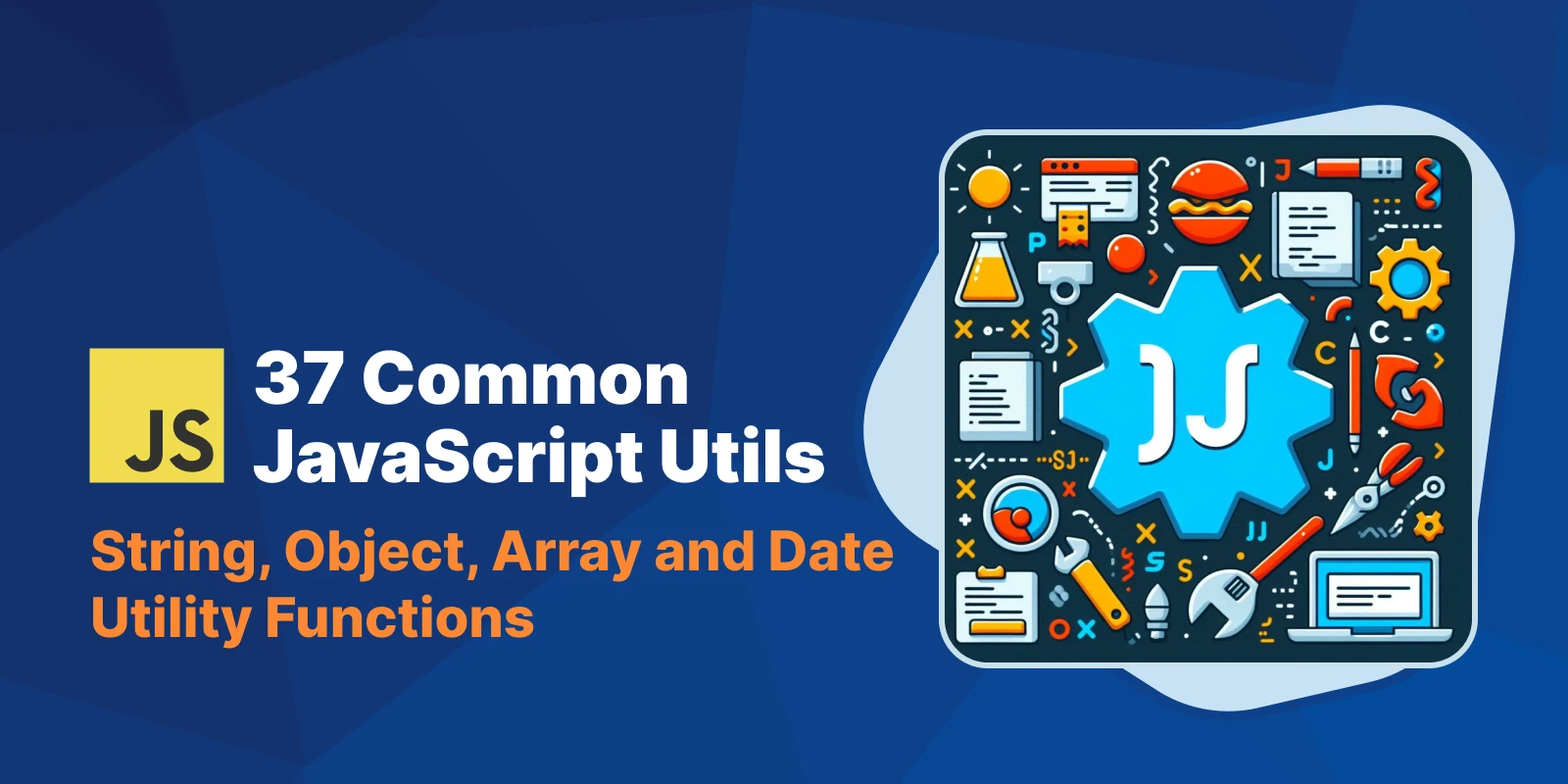
Utility functions are like shortcuts for your code. They let you handle things like arrays, dates, and strings without writing a lot of extra code. Instead of doing the same tasks over and over, you can use these functions to get the job done faster, consistent and prevent errors.
In this article, we’ll look at 37 common JavaScript utility functions that every developer should know. If you’re new to JavaScript or haven’t documented your own utility functions, this article is for you. By learning and using these utilities, you’ll save time, reduce errors, and gain a deeper understanding of how to write clean, reusable code.
Related posts
- 20 Lesser-known javascript features You probably dont know
- Javascript Array/Object Desctructurung Explained
So, let’s get started and see how these utilities can make your JavaScript coding easier and more effective.
Common String Utils in Javascript
1. Capitalize
Capitalizes the first letter of a string.
function capitalize(str) {
return str.charAt(0).toUpperCase() + str.slice(1);
}
// Example usage
console.log(capitalize('hello')); // 'Hello'
2. Convert to Camel Case
Converts a string with hyphens to camel case (camelCase).
function toCamelCase(input) {
return (
input
// Replace kebab-case, snake_case, and spaces with a space
.replace(/[-_\s]+(.)?/g, (_, char) => (char ? char.toUpperCase() : ''))
// Handle PascalCase
.replace(/^[A-Z]/, (char) => char.toLowerCase())
);
}
// Test cases
console.log(toCamelCase('PascalCase')); // pascalCase
console.log(toCamelCase('kebab-case-string')); // kebabCaseString
console.log(toCamelCase('snake_case_string')); // snakeCaseString
console.log(toCamelCase('string with spaces')); // stringWithSpaces
3. Convert to Kebab Case
Converts a string to kebab case (kebab-case).
function toKebabCase(input) {
return (
input
// Handle camelCase and PascalCase by inserting a dash before uppercase letters
.replace(/([a-z])([A-Z])/g, '$1-$2')
// Replace underscores and spaces with dashes
.replace(/[_\s]+/g, '-')
// Convert the entire string to lowercase
.toLowerCase()
);
}
// Test cases
console.log(toKebabCase('camelCase')); // camel-case
console.log(toKebabCase('PascalCase')); // pascal-case
console.log(toKebabCase('snake_case_string')); // snake-case-string
console.log(toKebabCase('string with spaces')); // string-with-spaces
4. Convert to Snake Case
Converts a string to snake case (snake_case).
function toSnakeCase(input) {
return (
input
// Handle camelCase and PascalCase by inserting an underscore before uppercase letters
.replace(/([a-z])([A-Z])/g, '$1_$2')
// Replace dashes and spaces with underscores
.replace(/[-\s]+/g, '_')
// Convert the entire string to lowercase
.toLowerCase()
);
}
// Test cases
console.log(toSnakeCase('camelCase')); // camel_case
console.log(toSnakeCase('PascalCase')); // pascal_case
console.log(toSnakeCase('kebab-case-string')); // kebab_case_string
console.log(toSnakeCase('string with spaces')); // string_with_spaces
5. Convert to Pascal Case
Converts a string to pascal case (PascalCase).
function toPascalCase(input) {
return (
input
// Replace camelCase, kebab-case, snake_case, and spaces with a space
.replace(/([a-z])([A-Z])|[-_\s]+(.)?/g, (match, p1, p2, p3) => {
if (p2) {
return p1 + ' ' + p2;
}
return p3 ? ' ' + p3.toUpperCase() : '';
})
// Split by space, capitalize first letter of each word, and join
.split(' ')
.map((word) => word.charAt(0).toUpperCase() + word.slice(1).toLowerCase())
.join('')
);
}
// Test cases
console.log(toPascalCase('camelCase')); // CamelCase
console.log(toPascalCase('kebab-case-string')); // KebabCaseString
console.log(toPascalCase('snake_case_string')); // SnakeCaseString
console.log(toPascalCase('string with spaces')); // StringWithSpaces
6. Check if String Contains Another String
Checks if a string contains a specified substring.
function contains(str, substring) {
return str.indexOf(substring) !== -1;
}
// Example usage
console.log(contains('Hello World', 'World')); // true
7. Replace All Occurrences
Replaces all occurrences of a substring within a string.
function replaceAll(str, find, replace) {
return str.split(find).join(replace);
}
// Example usage
console.log(replaceAll('Hello World', 'o', 'a')); // 'Hella Warld'
8. Count Occurrences of a Character
Counts the number of times a character appears in a string.
function countOccurrences(str, char) {
return str.split(char).length - 1;
}
// Example usage
console.log(countOccurrences('Hello World', 'o')); // 2
9. Escape HTML
Escapes HTML special characters to prevent XSS attacks.
function escapeHTML(str) {
return str.replace(/[&<>"']/g, function (match) {
const escapeChars = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
};
return escapeChars[match];
});
}
// Example usage
console.log(escapeHTML('<div>"Hello" & \'World\'</div>'));
// '<div>"Hello" & 'World'</div>'
10. Unescape HTML
Unescapes HTML special characters.
function unescapeHTML(str) {
return str.replace(/&|<|>|"|'/g, function (match) {
const unescapeChars = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
''': "'"
};
return unescapeChars[match];
});
}
// Example usage
console.log(unescapeHTML('<div>"Hello" & 'World'</div>'));
// '<div>"Hello" & \'World\'</div>'
11. Format Money
Formats a number as a currency string, with commas as thousands separators.
function formatMoney(amount, decimalCount = 2, decimal = '.', thousands = ',') {
try {
// Ensure the amount is a number and fix the decimal places
decimalCount = Math.abs(decimalCount);
decimalCount = isNaN(decimalCount) ? 2 : decimalCount;
const negativeSign = amount < 0 ? '-' : '';
let i = parseInt((amount = Math.abs(Number(amount) || 0).toFixed(decimalCount))).toString();
let j = i.length > 3 ? i.length % 3 : 0;
return (
negativeSign +
(j ? i.substr(0, j) + thousands : '') +
i.substr(j).replace(/(\d{3})(?=\d)/g, '$1' + thousands) +
(decimalCount
? decimal +
Math.abs(amount - i)
.toFixed(decimalCount)
.slice(2)
: '')
);
} catch (e) {
console.log(e);
}
}
// Test cases
console.log(formatMoney(1234567.89)); // 1,234,567.89
console.log(formatMoney(1234567.89, 3)); // 1,234,567.890
console.log(formatMoney(-1234567.89, 2, '.', ',')); // -1,234,567.89
console.log(formatMoney(1234567.89, 0, '.', ',')); // 1,234,568
console.log(formatMoney(1234.56, 2, ',', '.')); // 1.234,56
12. Truncate Paragraphs
Truncates a paragraph to a specified length and adds an ellipsis if truncated.
function truncateParagraph(paragraph, maxLength) {
if (paragraph.length <= maxLength) {
return paragraph;
}
return paragraph.slice(0, maxLength) + '...';
}
// Example usage
const paragraph =
'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent vitae eros eget tellus tristique bibendum. Donec rutrum sed sem quis venenatis.';
console.log(truncateParagraph(paragraph, 50));
// 'Lorem ipsum dolor sit amet, consectetur adipiscing...'
console.log(truncateParagraph(paragraph, 100));
// 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Praesent vitae eros eget tellus tristique b...'
If you want truncate string for styling purpose, you can do this with Tailwindcss truncate / line-clamp
Common Object Utils in Javascript
13. Check if a value is an object
Checks if a value is an object.
function isObject(value) {
return value !== null && typeof value === 'object' && !Array.isArray(value);
}
console.log(isObject({ a: 1 })); // true
console.log(isObject([1, 2, 3])); // false
14. Merge Objects (Flat object)
Merges two or more objects into one.
function mergeObjects(...objs) {
return Object.assign({}, ...objs);
}
console.log(mergeObjects({ a: 1 }, { b: 2 }, { a: 3 })); // { a: 3, b: 2 }
15. Deep Merge Objects
Deeply merges two or more objects.
function deepMerge(...objects) {
const isObject = (obj) => obj && typeof obj === 'object';
return objects.reduce((prev, obj) => {
Object.keys(obj).forEach((key) => {
const prevVal = prev[key];
const objVal = obj[key];
if (Array.isArray(prevVal) && Array.isArray(objVal)) {
prev[key] = prevVal.concat(...objVal);
} else if (isObject(prevVal) && isObject(objVal)) {
prev[key] = deepMerge(prevVal, objVal);
} else {
prev[key] = objVal;
}
});
return prev;
}, {});
}
const obj1 = { a: 1, b: { x: 10 } };
const obj2 = { b: { y: 20 }, c: 3 };
console.log(deepMerge(obj1, obj2)); // { a: 1, b: { x: 10, y: 20 }, c: 3 }
16. Deep Clone object
Creates a deep clone of an object.
function deepClone(obj) {
return JSON.parse(JSON.stringify(obj));
}
const original = { a: 1, b: { c: 2 } };
const clone = deepClone(original);
clone.b.c = 3;
console.log(original.b.c); // 2
console.log(clone.b.c); // 3
Check The Right Way to Clone Nested Object/Array in Javascript for more advanced use cases.
17. Omit / Delete keys from object
Returns a new object with specified keys omitted.
function omitKeys(obj, keys) {
const result = { ...obj };
keys.forEach((key) => delete result[key]);
return result;
}
console.log(omitKeys({ a: 1, b: 2, c: 3 }, ['a', 'c'])); // { b: 2 }
18. Pick keys to create new object
Returns a new object with only the specified keys.
function pickKeys(obj, keys) {
const result = {};
keys.forEach((key) => {
if (obj.hasOwnProperty(key)) {
result[key] = obj[key];
}
});
return result;
}
console.log(pickKeys({ a: 1, b: 2, c: 3 }, ['a', 'c'])); // { a: 1, c: 3 }
19. Invert Object keys and values
Inverts the keys and values of an object.
function invertObject(obj) {
const result = {};
Object.keys(obj).forEach((key) => {
result[obj[key]] = key;
});
return result;
}
console.log(invertObject({ a: 1, b: 2, c: 3 })); // { '1': 'a', '2': 'b', '3': 'c' }
20. Check if object is empty
Checks if an object is empty (has no enumerable properties).
function isEmptyObject(obj) {
return Object.keys(obj).length === 0;
}
console.log(isEmptyObject({})); // true
console.log(isEmptyObject({ a: 1 })); // false
21. Map Object
Creates a new object with the same keys but with values transformed by a function. Similar to array.map()
, but for object
function mapObject(obj, fn) {
const result = {};
Object.keys(obj).forEach((key) => {
result[key] = fn(obj[key], key);
});
return result;
}
console.log(mapObject({ a: 1, b: 2, c: 3 }, (value) => value * 2)); // { a: 2, b: 4, c: 6 }
22. Filter object
Creates a new object with only the properties that pass a filter function. Similar to array.filter()
, but for object
function filterObject(obj, fn) {
const result = {};
Object.keys(obj).forEach((key) => {
if (fn(obj[key], key)) {
result[key] = obj[key];
}
});
return result;
}
console.log(filterObject({ a: 1, b: 2, c: 3 }, (value) => value > 1)); // { b: 2, c: 3 }
23. Freeze Object
Freezes an object, making it immutable.
function freezeObject(obj) {
return Object.freeze(obj);
}
const obj = { a: 1, b: 2 };
const frozenObj = freezeObject(obj);
frozenObj.a = 3; // Will not change the value
console.log(frozenObj.a); // 1
24. Deep Freeze Object
Deeply freezes an object, making it and all nested objects immutable.
function deepFreeze(obj) {
Object.keys(obj).forEach((name) => {
const prop = obj[name];
if (typeof prop == 'object' && prop !== null) {
deepFreeze(prop);
}
});
return Object.freeze(obj);
}
const obj = { a: 1, b: { c: 2 } };
deepFreeze(obj);
obj.b.c = 3; // Will not change the value
console.log(obj.b.c); // 2
Common Array Utils in Javascript
25. Group Array Based on specified function
Groups the elements of an array based on a specified function.
function groupBy(array, fn) {
const map = new Map();
array.forEach((item) => {
const key = fn(item);
const collection = map.get(key);
if (!collection) {
map.set(key, [item]);
} else {
collection.push(item);
}
});
return map;
}
const people = [
{ name: 'Alice', age: 21 },
{ name: 'Bob', age: 22 },
{ name: 'Charlie', age: 21 }
];
const groupedByAge = groupBy(people, (person) => person.age);
console.log(groupedByAge);
// Map { 21 => [{ name: 'Alice', age: 21 }, { name: 'Charlie', age: 21 }], 22 => [{ name: 'Bob', age: 22 }] }
26. Array Intersection
Returns the intersection of two arrays.
function intersect(array1, array2) {
return array1.filter((x) => array2.includes(x));
}
const array1 = [1, 2, 3, 4];
const array2 = [3, 4, 5, 6];
console.log(intersect(array1, array2)); // [3, 4]
27. Zip Arrays
Combines two arrays into an array of pairs.
function zip(array1, array2) {
return array1.map((value, index) => [value, array2[index]]);
}
const array1 = [1, 2, 3];
const array2 = ['a', 'b', 'c'];
console.log(zip(array1, array2)); // [[1, 'a'], [2, 'b'], [3, 'c']]
28. Remove duplicate item
Removes duplicate values from an array.
function uniqueArray(arr) {
return [...new Set(arr)];
}
console.log(uniqueArray([1, 2, 2, 3, 4, 4, 5])); // [1, 2, 3, 4, 5]
29. Split array
Splits an array into chunks of specified size.
function chunkArray(arr, size) {
const result = [];
for (let i = 0; i < arr.length; i += size) {
result.push(arr.slice(i, i + size));
}
return result;
}
console.log(chunkArray([1, 2, 3, 4, 5], 2)); // [[1, 2], [3, 4], [5]]
30. Array difference
Returns the difference between two arrays.
function arrayDifference(arr1, arr2) {
return arr1.filter((item) => !arr2.includes(item));
}
console.log(arrayDifference([1, 2, 3], [2, 3, 4])); // [1]
Common Date Utils in Javascript
These utility functions can help you perform various date manipulations and formatting tasks in JavaScript efficiently.
31. Format date without library
Formats a date into a specified string format.
function formatDate(date, format) {
const map = {
mm: ('0' + (date.getMonth() + 1)).slice(-2),
dd: ('0' + date.getDate()).slice(-2),
yyyy: date.getFullYear(),
hh: ('0' + date.getHours()).slice(-2),
MM: ('0' + date.getMinutes()).slice(-2),
ss: ('0' + date.getSeconds()).slice(-2)
};
return format.replace(/mm|dd|yyyy|hh|MM|ss/gi, (matched) => map[matched]);
}
const date = new Date();
console.log(formatDate(date, 'dd-mm-yyyy hh:MM:ss')); // Example: 28-07-2024 14:30:45
32. Add days
Adds a specified number of days to a date.
function addDays(date, days) {
const result = new Date(date);
result.setDate(result.getDate() + days);
return result;
}
const date = new Date();
console.log(addDays(date, 5)); // Adds 5 days to the current date
33. Substract days
Subtracts a specified number of days from a date.
function subtractDays(date, days) {
const result = new Date(date);
result.setDate(result.getDate() - days);
return result;
}
const date = new Date();
console.log(subtractDays(date, 5)); // Subtracts 5 days from the current date
34. Count days between 2 dates
Calculates the number of days between two dates.
function daysBetween(date1, date2) {
const oneDay = 24 * 60 * 60 * 1000; // hours * minutes * seconds * milliseconds
const diffDays = Math.round(Math.abs((date2 - date1) / oneDay));
return diffDays;
}
const date1 = new Date('2024-07-01');
const date2 = new Date('2024-07-28');
console.log(daysBetween(date1, date2)); // Example: 27 days
35. Check if a date is weekend
Checks if a given date falls on a weekend.
function isWeekend(date) {
const day = date.getDay();
return day === 0 || day === 6;
}
const date = new Date();
console.log(isWeekend(date)); // Returns true if the date is a weekend, otherwise false
36. Count days of a month
Gets the number of days in a specific month of a specific year.
function getDaysInMonth(month, year) {
return new Date(year, month + 1, 0).getDate();
}
const month = 6; // July
const year = 2024;
console.log(getDaysInMonth(month, year)); // Example: 31 days in July 2024
37. Duration between 2 dates
function getDuration(startDate, endDate) {
const diff = endDate - startDate;
return Math.floor(diff / (1000 * 60 * 60));
// 1000 * 60 * 60 for duration in hours
// 1000 * 60 * 60 * 24 for duration in days
// 1000 * 60 for duration in minutes
}
const startDate = new Date('2024-07-01T12:00:00');
const endDate = new Date('2024-07-01T15:30:00');
console.log(getDurationInHours(startDate, endDate)); // Duration in hours
For more advance use-cases and tested utils for Date, consider using date library like day.js
Conclusion
We’ve covered 37 useful JavaScript utility functions that can help you code more efficiently. These functions simplify tasks like array handling, date formatting, and string manipulation. Using them can save you time and reduce errors in your code.
If this article was helpful, please share it with others who might benefit from it. Bookmark it for future reference, and if you have any favorite utility functions that weren’t mentioned, feel free to share them in the comments.
Happy coding!